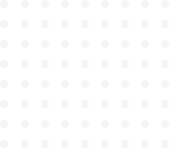
Tailwind Crash Course - Project Based Learning
Flexbox Wrap
Alright, one last thing I want to talk about in flex is FLEXBOX WRAP.
Let’s take a look at what would happen if we have a lot of items. Let’s create a regular flex container using just .flex
and add multiple boxes under it.
<html>
<head>
<link href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css" rel="stylesheet">
</head>
<body>
<div class="h-screen flex">
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
</div>
</body>
</html>
And the output is below:
⚡ If you notice that it is not wrapping and not even concerning box width. Everything just got crunch together. So, in-comes flex wrap.
Wrap Modes | |
no-wrap wrap wrap-reverse |
Default |
As you can see, .flex-no-wrap
is the default but we also have .flex-wrap
and .flex-wrap-reverse
. Let’s take a look at that.
Simply adding .flex-wrap
in container div.
<html>
<head>
<link href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css" rel="stylesheet">
</head>
<body>
<div class="h-screen flex flex-wrap"> <!-- adding .flex-wrap class-->
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
</div>
</body>
</html>
In the output, you can see that we are now able to wrap this all the way around and it doesn’t matter how many we have.
Now the next thing here is if we resize it, see below! How it changes.
Okay! Now you also have .flex-wrap-reverse
. Let’s change .flex-wrap
to .flex-wrap-reverse
in container div.
<html>
<head>
<link href="https://unpkg.com/tailwindcss@^1.0/dist/tailwind.min.css" rel="stylesheet">
</head>
<body>
<div class="h-screen flex flex-wrap-reverse"> <!-- .flex-wrap-reverse class-->
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
<div class="bg-yellow-600 w-16 h-16">1</div>
<div class="bg-teal-700 w-16 h-16">2</div>
<div class="bg-red-700 w-16 h-16">3</div>
</div>
</body>
</html>
At a first glance, It may not be super obvious what it actually doing. But notice that, it’s still in the right direction. It’s not flipping the direction of the wrapping. Notice that we still have very bottom 1-2-3-1 and then it jumps up 1 row: 2-3-1-2-3. So that’s what it is doing. It simply just reversing the order of the rows.
So the last row is the first row and the second to the last is your second row and so on and so forth.