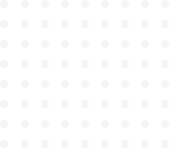
ReactJS project based training with Road Trip Planner App
Understanding the project directory
In the last article on Project setup, we discussed the root directory, now we’ll go inside the /public and /src directories.
Public folder
favicon.ico
This is your logo or icon image which appears on the left-hand side of your browser tab. You can replace it with your own icon, but do remember to delete this one and save yours under the same name.
For the purpose of this project, I have collected all the images, SVGs, or illustrations inside an asset folder which you can find on our GitHub repository, so for favicon, you just copy and paste it inside your public folder
manifest.json
It’s your react web app’s manifest file which consists of information about the web app like logo images, and other descriptions like author’s name, etc., here you will find information about icons like source, size, etc.
For the sake of simplicity, I have kept just one size for the favicon image in my manifest file,
{
"short_name": "React App",
"name": "Create React App Sample",
"icons": [
{
"src": "favicon.ico",
"sizes": "64x64 32x32 24x24 16x16",
"type": "image/x-icon"
}
],
"start_url": ".",
"display": "standalone",
"theme_color": "#000000",
"background_color": "#ffffff"
}
index.html
Remember from the introduction article, your react code needs to access the DOM in order to show up on the webpage, index.html file is that access. When webpack bundles all of your scripts/javascript files into a single file, it injects this bundled file inside index.html. So basically, index.html provides a context to the react code to render to.
Source folder (src)
This is where we are going to store all of the scripts and styles of your project along with the assets used. But first, you just delete all of these files inside the src folder
đŸ‘† Default structure
Now your src directory should look like this, 3 folders (assets, components, and pages), 2 files (app.js and index.js)
đŸ‘†This is how we are going to organize it.
Assets
Here we will curate all the assets(basically static files) like images and SVGs etc to be used in the project. For this project, you can copy the content from the GitHub repository, paste it in here, and your assets folder should look like this,
index.js file to import and export
Why?: Whenever you have multiple files in a directory to export(which is usually the case), it’s really convenient to use the index.js file as a single point of export for a directory.
How?: Whenever you put an index.js file inside a folder directory/folder, the whole directory points to this index.js file, ( by default in javascript a directory points to its index.js file, like it will be treated like an index for the whole directory)
For example in this case, whenever you need to import an SVG or jpg file from assets inside a src/file, you don’t need to mention the complete path of this file in your import statement, like import { explore } from “../assets/explore.svg”
, you can instead import { explore, trip, destination, throughthedesert } from “../assets”
, because the assets directory points to index.js inside it, and the index.js file contains exports of all these files like,
import destination from "./destination.svg";
import explore from "./explore.svg";
import throughthedesert from "./throughthedesert.svg";
import trip from "./trip.svg";
import udaipur from "./udaipur.jpg";
const svgs = {
destination,
trip,
explore,
throughthedesert,
udaipur
}
export default svgs;
đŸ‘† index.js inside assets/
Action Item: Create a folder named assets under the src directory and copy all the assets from the project repo here. Create the above index.js file if you wish.
Components folder
From the intro article, you might remember that components are the pieces that collectively make a react application.
In this folder we collect all the main components to be used in our app, these components are to be exported to their respective pages then. Here also you can create an index.js file importing all four components into this file and then exporting them as an object just like we did in the assets folder.
Action Item: under the components folder, create the following component files, and leave them empty for now.
Pages
SPAs and React
React is meant for single-page applications, you must have heard it at least once if you’ve done any research on react, but when first I got to know about this concept, I was confused, like why? I mean I had always seen many multipage applications and also built a few by then, but I couldn’t make sense of it until I spent some time reading on SPAs, but you don’t have to go through that confusion hole, lemme explain real quick,
In ReactJS whenever you move across different pages, the URL of the page doesn’t change completely, changing the complete URL would typically mean loading a new HTML file and rewriting the whole web page again from the scratch, but you must have noticed we’ve got just one index.html file in our project directory. So what’s happening here is, that when you navigate to a different page on a react application, react just loads new content into the same page and adds a subroute to the same URL thus making it feel like a new page.
So in react a new page is just a new page component inserted into the same HTML file. Remember the root element from the Rendering section of the previous article, it’s where the new page content is loaded, we’ll see it in detail later.
Right now our app consists of two pages, - a home page and a planning page which
-
Home Page
-
Plan Page
These pages are also just react components, but to make them appear as separate pages, we need to make them subroutes under the main app component, for that, we’ll use a dependency called React-router.
Action Item: Under page, folder create empty page files (with .jsx extension) and their css files (with .css extension), like this,
Now that we have the project directory ready, let’s get to the building now,
app.js
This is the file where we conclude our entire app, add routes, etc. Just to organize our website better we are going to import this app component into another file index.js which acts as the entry point for our react application.
Let’s start with a functional component, for now, to keep it simple, we just have a simple text on our page,
import React from "react";
const App = () => {
return <p>
This is a roadtrip app.
</p>
}
export default App;
Index.js:
I must have mentioned before that webpack(module bundler) is configured to consider index.js file as the entry point of our application, meaning that it begins with this index.js file to bundle all of the javascript files of your app and creates a single bundle.js file. Webpack then injects this bundle.js file into the HTML file (index.html).
Inside index.js file, we connect our react code to the HTML or DOM.
Inside this file we need to achieve the following,
-
access
<div id="root"></div>
the root element of index.html from the react code -
render the app component inside this root element
ReactDOM is the library that exists at the top of our react-app connecting react application code to the actual DOM, when we are done creating the components and the structure of our app, we want to insert/render this code inside the actual DOM, for this we need ReactDOM.
createRoot
method is used to create a root object by passing the reference to the root DOM element, using the render
method of this root object we write (or replace) the content of <div id="root"></div>
Here, <App/>
is inserted inside <div id="root"></div>
.
import ReactDOM from "react-dom/client";
import React from "react";
import App from "./app";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(<App />);
Now run the react-script npm run start inside a new terminal to run the development server, it’ll open a localhost page like this on your default browser,
Whenever you edit any file inside your project, on save it will automatically reflect on the web page.
So the top-level container is ready for our app, now we need to create other components that go inside this app component, you should keep observing how we will build this app using a mix of top-down and bottom-up approaches on the component tree.